Computer Systems 5th Edition by Stanley Warford – Ebook PDF Instant Download/Delivery: 1284079635 ,9781284079630
Full dowload Computer Systems 5th Edition after payment
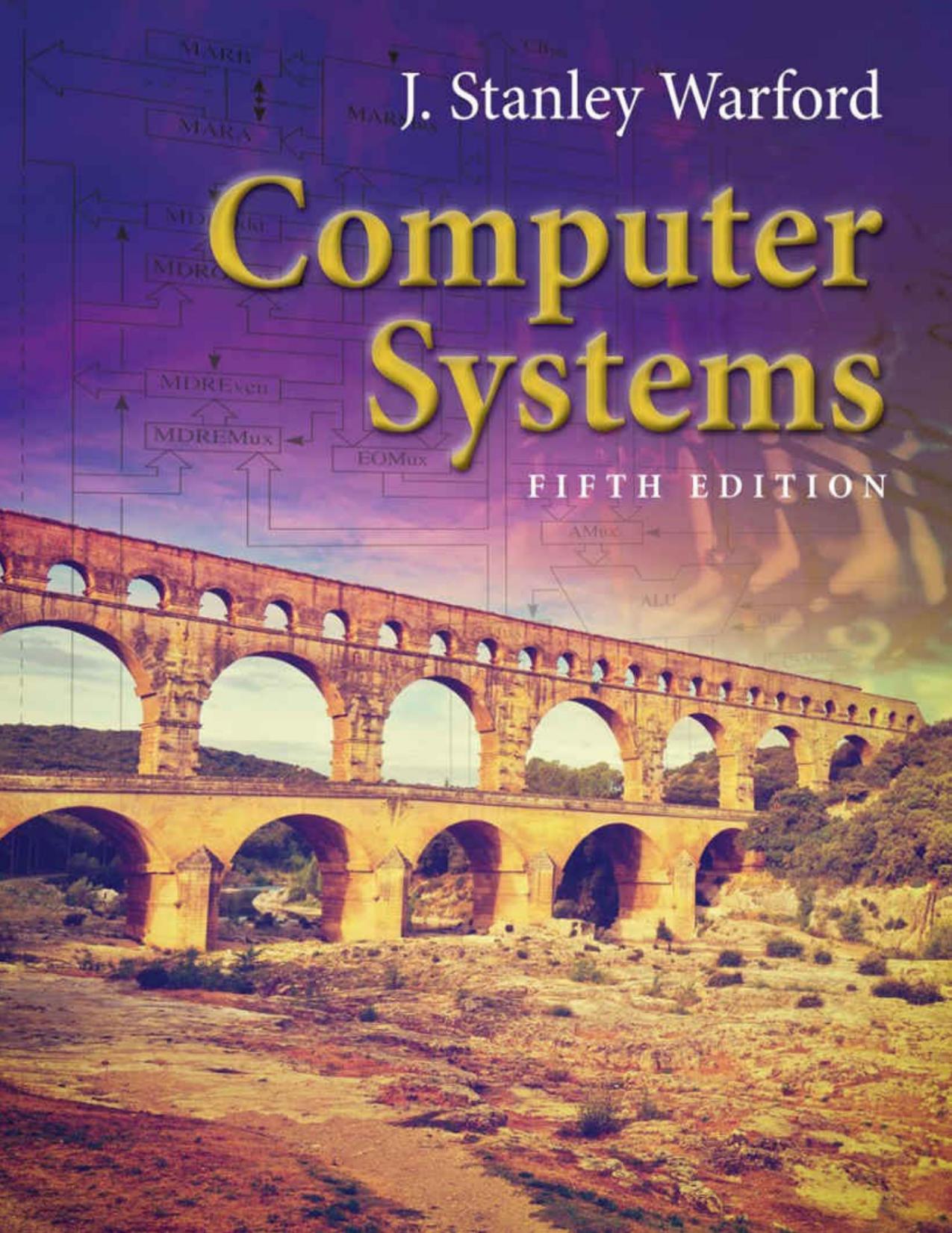
Product details:
ISBN 10: 1284079635
ISBN 13: 978-1284079630
Author: Stanley Warford
Computer Systems, Fifth Edition provides a clear, detailed, step-by-step introduction to the central concepts in computer organization, assembly language, and computer architecture. It urges students to explore the many dimensions of computer systems through a top-down approach to levels of abstraction. By examining how the different levels of abstraction relate to one another, the text helps students look at computer systems and their components as a unified concept. New & Key Features: – New high-order language ― The high-order language is changed from C++ to C. The C language is more common as a systems programming language and is more appropriate for a computer systems text. – New sidebars ― Each sidebar is a real-world example of the concepts described in that chapter. As most of the chapters describe the Pep/9 virtual machine, the sidebars for those chapters show corresponding implementations for the Intel x86 architecture. – New and expanded topics ― New and expanded topics include, QR codes, color displays, Unicode, UTF-32 and UTF-8 encoding, floating point underflow, big-endian and little-endian order, memory alignment issues, and expanded RISC design principles and MIPS coverage to contrast with the Pep/9 CISC design. – New virtual machine ― Pep/8, the virtual machine for the two previous editions, is now superseded by the new and improved Pep/9. Pep/9 retains the same eight addressing modes of Pep/8 but now includes memory-mapped I/O, an improved return-from-subroutine instruction, a new native compare-byte instruction, improved instruction mnemonics, and a new hexadecimal output trap instruction. – New software ― The Pep/9 system in the text is supported by two updated open source software applications, the assembler/simulator and the CPU simulator.
Computer Systems 5th Table of contents:
Level 7 Application
1. Computer Systems
1.1 Levels of Abstraction
Abstraction in Art
Abstraction in Documents
Abstraction in Organizations
Abstraction in Machines
Abstraction in Computer Systems
1.2 Hardware
Central Processing Unit
Main Memory
Disk
1.3 Software
Operating Systems
Software Analysis and Design
1.4 Digital Information
Quantifying Space
Quantifying Time
Quick Response Codes
Images
1.5 Database Systems
Relations
Queries
Structure of the Language
Chapter Summary
Exercises
Level 6 High-Order Language
2. C
2.1 Variables
The C Compiler
Machine Independence
The C Memory Model
Global Variables and Assignment Statements
Local Variables
2.2 Flow of Control
The If/Else Statement
The Switch Statement
The While Loop
The Do Loop
Arrays and the For Loop
2.3 Functions
Void Functions and Call-by-Value Parameters
Functions
Call-by-Reference Parameters
2.4 Recursion
A Factorial Function
Thinking Recursively
Recursive Addition
A Binomial Coefficient Function
Reversing the Elements of an Array
Towers of Hanoi
Mutual Recursion
The Cost of Recursion
2.5 Dynamic Memory Allocation
Pointers
Structures
Linked Data Structures
Chapter Summary
Exercises
Problems
Level 3 Instruction Set Architecture
3. Information Representation
3.1 Unsigned Binary Representation
Binary Storage
Integers
Base Conversions
Range for Unsigned Integers
Unsigned Addition
The Carry Bit
3.2 Two’s Complement Binary Representation
Two’s Complement Range
Base Conversions
The Number Line
The Overflow Bit
The Negative and Zero Bits
3.3 Operations in Binary
Logical Operators
Register Transfer Language
Arithmetic Operators
Rotate Operators
3.4 Hexadecimal and Character Representations
Hexadecimal
Base Conversions
ASCII Characters
Unicode Characters
3.5 Floating-Point Representation
Binary Fractions
Excess Representations
The Hidden Bit
Special Values
The IEEE 754 Floating-Point Standard
3.6 Models
Chapter Summary
Exercises
Problems
4. Computer Architecture
4.1 Hardware
Central Processing Unit (CPU)
Main Memory
Input/Output Devices
Data and Control
Instruction Format
4.2 Direct Addressing
The Stop Instruction
The Load Word Instruction
The Store Word Instruction
The Add Instruction
The Subtract Instruction
The And and Or Instructions
The Invert and Negate Instructions
The Load Byte and Store Byte Instructions
The Input and Output Devices
Big Endian Versus Little Endian
4.3 von Neumann Machines
The von Neumann Execution Cycle
A Character Output Program
von Neumann Bugs
A Character Input Program
Converting Decimal to ASCII
A Self-Modifying Program
4.4 Programming at Level ISA3
Read-Only Memory
The Pep/9 Operating System
Using the Pep/9 System
Chapter Summary
Exercises
Problems
Level 5 Assembly
5. Assembly Language
5.1 Assemblers
Instruction Mnemonics
Pseudo-Operations
The .ASCII and .END Pseudo-ops
Assemblers
The .BLOCK Pseudo-op
The .WORD and .BYTE Pseudo-ops
Using the Pep/9 Assembler
Cross Assemblers
5.2 Immediate Addressing and the Trap Instructions
Immediate Addressing
The DECI, DECO, and BR Instructions
The STRO Instruction
Interpreting Bit Patterns: The HEXO Instruction
Disassemblers
5.3 Symbols
A Program with Symbols
A von Neumann Illustration
5.4 Translating from Level HOL6
The Printf() Function
Variables and Types
Global Variables and Assignment Statements
Type Compatibility
Pep/9 Symbol Tracer
The Shift and Rotate Instructions
Constants and .EQUATE
Placement of Instructions and Data
Chapter Summary
Exercises
Problems
6. Compiling to the Assembly Level
6.1 Stack Addressing and Local Variables
Stack-Relative Addressing
Accessing the Run-Time Stack
Local Variables
6.2 Branching Instructions and Flow of Control
Translating the If Statement
Optimizing Compilers
Translating the If/Else Statement
Translating the While Loop
Translating the Do Loop
Translating the For Loop
Spaghetti Code
Flow of Control in Early Languages
The Structured Programming Theorem
The Goto Controversy
6.3 Function Calls and Parameters
Translating a Function Call
Translating Call-by-Value Parameters with Global Variables
Translating Call-by-Value Parameters with Local Variables
Translating Non-void Function Calls
Translating Call-by-Reference Parameters with Global Variables
Translating Call-by-Reference Parameters with Local Variables
Translating Boolean Types
6.4 Indexed Addressing and Arrays
Translating Global Arrays
Translating Local Arrays
Translating Arrays Passed as Parameters
Translating the Switch Statement
6.5 Dynamic Memory Allocation
Translating Global Pointers
Translating Local Pointers
Translating Structures
Translating Linked Data Structures
Chapter Summary
Exercises
Problems
7. Language Translation Principles
7.1 Languages, Grammars, and Parsing
Concatenation
Languages
Grammars
A Grammar for C Identifiers
A Grammar for Signed Integers
A Context-Sensitive Grammar
The Parsing Problem
A Grammar for Expressions
A C Subset Grammar
Context Sensitivity of C
7.2 Finite-State Machines
An FSM to Parse an Identifier
Simplified FSMs
Nondeterministic FSMs
Machines with Empty Transitions
Multiple Token Recognizers
Grammars Versus FSMs
7.3 Implementing Finite-State Machines
The Compilation Process
A Table-Lookup Parser
A Direct-Code Parser
An Input Buffer Class
A Multiple-Token Parser
7.4 Code Generation
A Language Translator
Parser Characteristics
Chapter Summary
Exercises
Problems
Level 4 Operating System
8. Process Management
8.1 Loaders
The Pep/9 Operating System
The Pep/9 Loader
Program Termination
8.2 Traps
The Trap Mechanism
The RETTR Instruction
The Trap Handlers
Trap Addressing Mode Assertion
Trap Operand Address Computation
The No-Operation Trap Handlers
The DECI Trap Handler
The DECO Trap Handler
The HEXO and STRO Trap Handlers and Operating System Vectors
8.3 Concurrent Processes
Asynchronous Interrupts
Processes in the Operating System
Multiprocessing
A Concurrent Processing Program
Critical Sections
A First Attempt at Mutual Exclusion
A Second Attempt at Mutual Exclusions
Peterson’s Algorithm for Mutual Exclusion
Semaphores
Critical Sections with Semaphores
8.4 Deadlocks
Resource Allocation Graphs
Deadlock Policy
Chapter Summary
Exercises
Problems
9. Storage Management
9.1 Memory Allocation
Uniprogramming
Fixed-Partition Multiprogramming
Logical Addresses
Variable-Partition Multiprogramming
Paging
9.2 Virtual Memory
Large Program Behavior
Virtual Memory
Demand Paging
Page Replacement
Page-Replacement Algorithms
9.3 File Management
Disk Drives
File Abstraction
Allocation Techniques
9.4 Error-Detecting and Error-Correcting Codes
Error-Detecting Codes
Code Requirements
Single-Error-Correcting Codes
9.5 RAID Storage Systems
RAID Level 0: Nonredundant Striped
RAID Level 1: Mirrored
RAID Levels 01 and 10: Striped and Mirrored
RAID Level 2: Memory-Style ECC
RAID Level 3: Bit-Interleaved Parity
RAID Level 4: Block-Interleaved Parity
RAID Level 5: Block-Interleaved Distributed Parity
Chapter Summary
Exercises
Level 1 Logic Gate
10. Combinational Circuits
10.1 Boolean Algebra and Logic Gates
Combinational Circuits
Truth Tables
Boolean Algebra
Boolean Algebra Theorems
Proving Complements
Logic Diagrams
Alternate Representations
10.2 Combinational Analysis
Boolean Expressions and Logic Diagrams
Truth Tables and Boolean Expressions
Two-Level Circuits
The Ubiquitous NAND
10.3 Combinational Design
Canonical Expressions
Three-Variable Karnaugh Maps
Four-Variable Karnaugh Maps
Dual Karnaugh Maps
Don’t-Care Conditions
10.4 Combinational Devices
Viewpoints
Multiplexer
Binary Decoder
Demultiplexer
Adder
Adder/Subtracter
Arithmetic Logic Unit
Abstraction at Level LG1
Chapter Summary
Exercises
11. Sequential Circuits
11.1 Latches and Clocked Flip-Flops
The SR Latch
The Clocked SR Flip-Flop
The Master–Slave SR Flip-Flop
The Basic Flip-Flops
The JK Flip-Flop
The D Flip-Flop
The T Flip-Flop
Excitation Tables
11.2 Sequential Analysis and Design
A Sequential Analysis Problem
Preset and Clear
Sequential Design
A Sequential Design Problem
11.3 Computer Subsystems
Registers
Buses
Memory Subsystems
Address Decoding
A Two-Port Register Bank
Chapter Summary
Exercises
Level 2 Microcode
12. Computer Organization
12.1 Constructing a Level-ISA3 Machine
The CPU Data Section
The von Neumann Cycle
The Store Byte Direct Instruction
Bus Protocols
The Store Word Direct Instruction
The Add Immediate Instruction
The Load Word Indirect Instruction
The Arithmetic Shift Right Instruction
The CPU Control Section
12.2 Performance
The Data Bus Width and Memory Alignment
Memory Alignment
The Definition of an n-Bit Computer
Cache Memories
The System Performance Equation
RISC Versus CISC
12.3 The MIPS Machine
The Register Set
The Addressing Modes
The Instruction Set
MIPS Computer Organization
Pipelining
12.4 Conclusion
Simplifications in the Model
The Big Picture
Chapter Summary
Exercises
Problems
People also search for Computer Systems 5th:
harris computer systems
computer systems analyst job description
arabic computer systems
a computer systems analyst mostly
computer systems bryant o’hallaron